3.2. While loop
Loops can be nested one into another, and at each iteration of the outer loop, all iterations of the inner loop have already been completed. For convenience, you can initialize the so-called “counter” variable before each definition of the loop, setting it to its initial value and increasing it at each iteration, as well as including this variable in the test expression. The loop will end if the expression fails.
- Start a new Python program by initializing a counter variable and defining an outer loop using that variable in the test expression.
I = 1
while i <4:
- Then add indented instructions to print out the counter value and increment its value at each iteration
of the loop.
print (‘\ nOuter Loop Iteration:’, i)
i + = 1
- Now (still indented) initialize the second counter variable and define the inner loop using that variable
in the test expression.
j = 1
while j <4:
- Finally, add further indented instructions to print the counter value and increment the counter value
with each iteration.
print (‘\ tInner Loop Iteration:’, j)
j + = 1
- Save the file in the working directory, open a command prompt and run the program – you will see the output at each iteration of the loop.
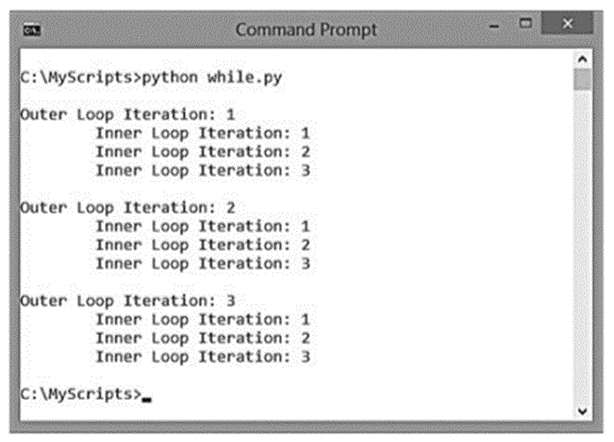