4.2. Working with buttons
- Start a new Python program with the gui module functions available and create a window with a title.
from tkinter import *
window = Tk ()
window.title (‘ButtonExample‘)
- 2. Now create a button that will exit the program.
btn_end = Button (window, text = ‘Close’, command = exit)
- Then add a function that will toggle the background color of the window after clicking another button.
deftog ():
if window.cget (‘bg‘) == ‘yellow’:
window.configure (bg = ‘gray’)
else:
window.configure (bg = ‘yellow’)
- 4. Now create a button that will invoke the function.
btn_tog = Button (window, text = ‘Switch’, command = tog)
- Add both buttons to the window, specifying horizontal and vertical fill options.
btn_end.pack (padx = 150, pady = 20)
btn_tog.pack (padx = 150, pady = 20)
- 6. Finally add the window event loop.
window.mainloop ()
- Save the file in your working directory, open a command prompt and run the program with the pythontk_button command. py – click the button to see the result of changing the background color of the window.
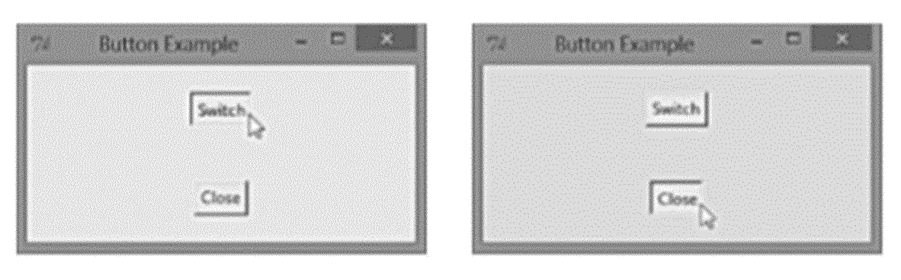