1.3 Getting to know the interpreter
The Python interpreter processes your program’s textual code and also has an interactive mode, useful for debugging and testing snippets of code. Python interactive mode can be accessed in several ways:
- from a normal command line – enter the python command to run the initial Python command line (symbols >>>), in which you interact with the interpreter;
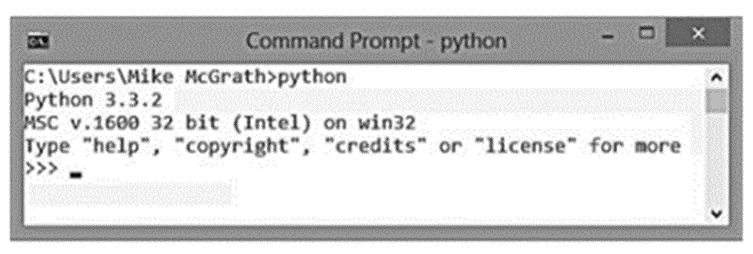