2.2 Assigning values
- Create a new program named Assignment containing the standard main method.
class Assignment
{
public static void main (String [] args) {}
}
- Inside the curly braces of the main method, add the following lines of code to add and assign a string value.
String txt = “Fantastic”;
String lang = “Java”;
txt + = lang; // Assignment with concatenated strings
System.out.println (“Adding and assigning lines:” + txt);
- 3. Add these lines for addition and assignment of integers.
int sum = 10;
int num = 20;
sum + = num; // Assign the result (10 + 20 = 30)
System.out.println (“Add and assign integers:” + sum);
- 4. Now add the lines that multiply and assign integers.
int factor = 5;
sum * = factor; // Assign the result (30 x 5 = 150)
System.out.println (“Result of multiplication” + sum);
- 5. Add lines that perform division and assignment of integers.
sum / = factor; // Assign the result (150 ÷ 5 = 30)
System.out.println (“Result of division:” + sum);
- 6. Save the program as Assignment.java, then compile and run.
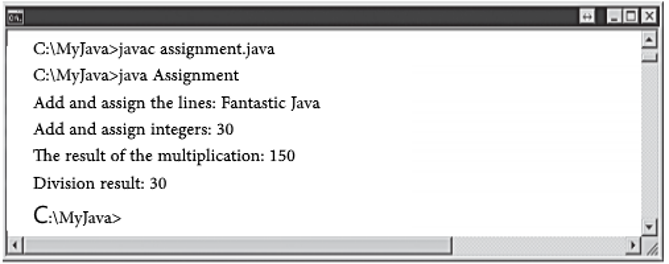